OpenAPI Generator + TypeScript
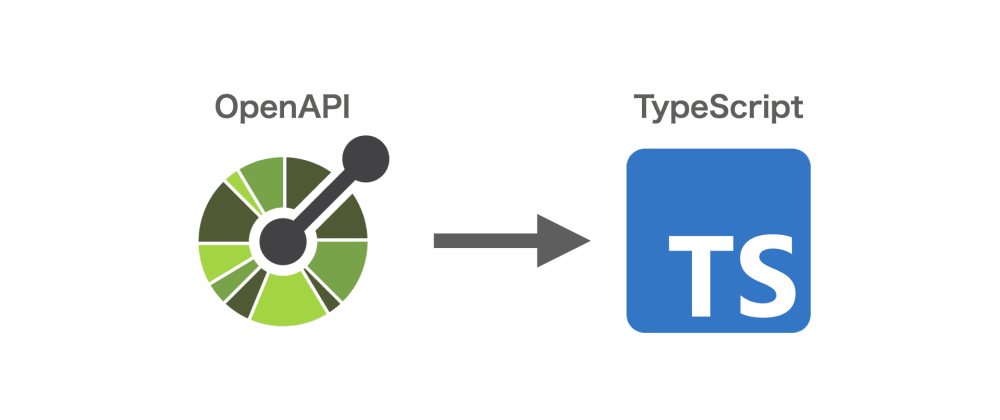
OpenApi generator là gì?
OpenAPI Generator là một nhánh của codegen swagger giữa các phiên bản 2.3.1 và 2.4.0. Rất nhiều bạn chỉ biết đến OpenAPI là 1 thư viện để viết tài liệu api cho phía backend. Những ngoài ra nó còn có thể tận dụng tài liệu OpenAPI phía backend (yaml file) để generate app client giúp cho việc call api phía client không có chút sai lệch nào so với backend. Điều này giúp cho việc chuẩn hóa giao tiếp giữa client vs server, việc giao tiếp 2 bên sẽ trở nên đơn giản, hiệu quả hơn.
OpenAPI được hỗ trợ tới hơn 50 client generators và hơn 40 ngôn ngữ khác nhau, điều này giúp cho nó khá dễ trong việc mở rộng. Để biết được nó sử dụng ở client như nào thì sau đây mình sẽ triển khai OpenAPI với vuejs nhé.
1. Cài đặt OpenAPI Generator CLI
npm install @ openapitools / openapi-generator-cli -g
or
yarn add -D @openapitools/openapi-generator-cli
2. Generate client
openapi-generator generate -g typescript-axios -i ./path/to/openapi.yaml -o ./frontend-application-directory/src/types/typescript-axios
Source được tạo ra sẽ có cấu trúc thư mục như sau:
./types
├── client-axios
│ ├── api.ts
│ ├── base.ts
│ ├── configuration.ts
│ ├── git_push.sh
│ └── index.ts
└── index.d.ts
3. Áp dụng vào vuejs
Ở đây mình sẽ áp dụng vào màn hình login nhé.
3.1 Yaml file và app client vừa tạo
Sau đây là thông tin loginId và password có trong api.ts được dùng để login. Dưới đây là file yaml và api.ts vừa được generate.
openapi.yaml
LoginRequest:
tags:
- Auth
title: LoginRequest
type: object
description: Thông tin xác thực
required: [loginId, password]
operationId: postAuthLogin
properties:
loginId:
type: string
description: ID đăng nhập
password:
type: string
description: Mật khẩu
types/typescript-client/api.ts
/**
* 認証情報
* @export
* @interface LoginRequest
*/
export interface LoginRequest {
/**
* ID đăng nhập
* @type {string}
* @memberof Auth
*/
loginId: string;
/**
* Mật khẩu
* @type {string}
* @memberof Auth
*/
password: string;
}
export class AuthApi extends BaseAPI {
public postAuthLogin(loginRequest: LoginRequest, options?: any) {
return AuthApiFp(this.configuration).postAuthLogin(loginRequest, options).then((request) => request(this.axios, this.basePath));
}
}
3.2. Sử dụng trong store(Vuex)
Ở đây mình sử dụng store để call api. File store của mình sẽ như sau:
import { Module, ActionTree, GetterTree, MutationTree } from 'vuex'
import { LoginRequest, AuthApi } from '@/types/typescript-axios'
import Cookies, { CookieSetOptions } from 'universal-cookie'
import { RootState } from '@/types/index'
interface AuthState {
}
interface LoginParams {
basePath?: string,
value: LoginRequest,
cookie: Cookies,
stage: string,
}
export const state = (): AuthState => ({
})
export const getters: GetterTree<AuthState, RootState> = {
}
export const mutations: MutationTree<AuthState> = {
}
export const actions: ActionTree<AuthState, RootState> = {
async login(_ctx, params: LoginParams) {
try {
const { data } = await(new AuthApi({ basePath: params.basePath }))
.postAuthLogin(params.value)
params.cookie.set(
'accessToken',
data.accessToken,
{
secure: params.stage !== 'local',
maxAge: data.expiresIn,
} as CookieSetOptions,
)
} catch (error) {
throw error.response
}
},
}
export const auth: Module<AuthState, RootState> = {
namespaced: true,
getters,
mutations,
actions,
}
Hy vọng bài viết của mình sẽ giúp các bạn có thể hiểu về OpenAPI hơn.
Chúc các bạn ngày càng phát triển bản thân hơn nhé!